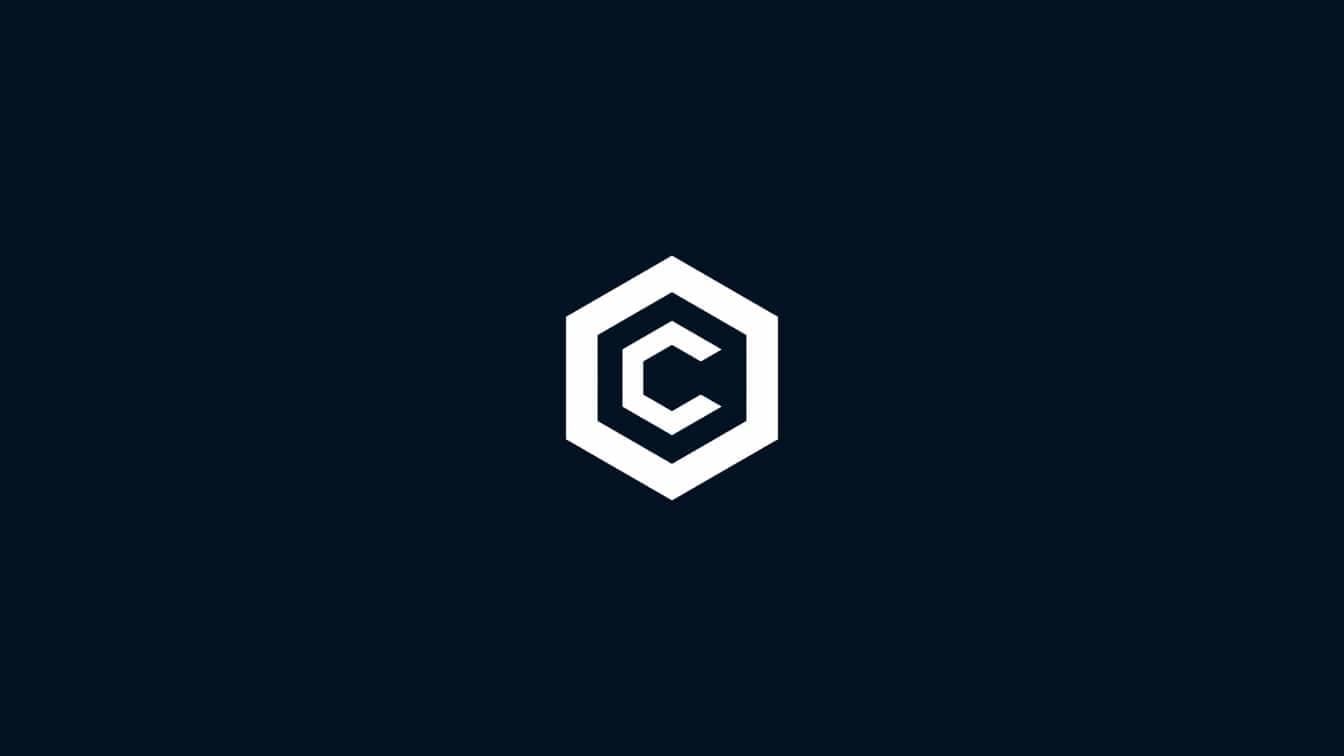
Australian entrepreneur and founder of Rubix Studios. Vincent specialises in branding, multimedia, and web development with a focus on digital innovation and emerging technologies.
Token creation on Cronos offers an efficient path for developers and organisations to participate in decentralised applications (dApps) and decentralised finance (DeFi). By leveraging the Ethereum-compatible Cronos blockchain, users can deploy ERC-20 tokens with low transaction costs and high scalability. This guide outlines the full process of creating and deploying an ERC-20 token using Remix IDE and MetaMask. It includes network setup, smart contract development, compilation, deployment, and interaction.
Prerequisites
Before proceeding, ensure the following:
- MetaMask: Installed and configured.
- Remix IDE: Accessible via Remix Ethereum IDE.

MetaMask
MetaMask is a browser extension wallet required to interact with Ethereum-compatible blockchains, including Cronos. If not already installed, download MetaMask from MetaMask.io.
Cronos
To configure MetaMask for the Cronos Mainnet:
- Open MetaMask and go to Settings > Networks > Add Network.
- Enter the following network details:
- Network Name: Cronos Mainnet
- New RPC URL: https://evm.cronos.org
- Chain ID: 25
- Currency Symbol: CRO
- Block Explorer URL: https://cronoscan.com
- Save the configuration and switch to Cronos Mainnet within MetaMask.
Once set up, MetaMask is ready to connect with Remix and deploy contracts on the Cronos blockchain.
Remix IDE
Remix IDE is a browser-based development environment designed for writing, compiling, and deploying smart contracts using the Solidity programming language.
Access
- Open Remix Ethereum IDE in a web browser.
- Allow required browser permissions if prompted.
Contract
- In the left sidebar, click the File Explorer icon.
- Select Create New File.
- Name the file MyToken.sol.
This file will hold the Solidity code for your ERC-20 token contract.
1// SPDX-License-Identifier: MIT2// Compatible with OpenZeppelin Contracts ^5.0.03pragma solidity ^0.8.27;45import { ERC20 } from "@openzeppelin/contracts/token/ERC20/ERC20.sol";6import { Ownable } from "@openzeppelin/contracts/access/Ownable.sol";78contract MyToken is ERC20, Ownable {9 constructor(address initialOwner)10 ERC20("MyToken", "MTK")11 Ownable(initialOwner)12 {13 _mint(msg.sender, 10_000_000 * 10 ** decimals());14 }1516 function mint(address to, uint256 amount) public onlyOwner {17 _mint(to, amount);18 }1920 function burn(uint256 amount) public {21 _burn(msg.sender, amount);22 }23}
Features
- Solidity version: ^0.8.27
- Token name: MyToken
- Symbol: MTK
- Supply: 10 million tokens minted at deployment
- Minting: Restricted to the contract owner
- Burning: Available to any token holder
- Security: Leverages OpenZeppelin’s audited ERC20 and Ownable implementations
This code ensures compatibility with the latest Solidity compiler and incorporates safe, production-ready patterns from the OpenZeppelin contract suite.
Explore our Einstein Contract on GitHub for more ideas on creating your smart contract. This resource includes advanced patterns for permission control, utility functions, and integration-ready modules.
Compile
- In Remix, go to the Solidity Compiler tab.
- Ensure the compiler version matches ^0.8.27.
- Click Compile MyToken.sol.
Deploy
- Open the Deploy & Run Transactions tab.
- Set environment to Injected Web3 to connect with MetaMask.
- Select the correct account in MetaMask.
- Choose MyToken from the contract dropdown.
- Click Deploy and confirm in MetaMask.

Verify
- After deployment, locate the contract address in Remix.
- To view the token in MetaMask:
- Click Add Token > Custom Token.
- Paste the contract address and complete the steps.
Interact
- Use the deployed instance in Remix to test functions such as mint or burn.
- Alternatively, interact through Cronoscan using your contract address.
You have successfully deployed an ERC-20 token on the Cronos blockchain using MetaMask and Remix IDE. The process included configuring MetaMask for the Cronos network, developing a standards-compliant smart contract using OpenZeppelin libraries, compiling, deploying, and confirming your contract on-chain.
This deployment provides a foundational asset for DeFi platforms, application integrations, or token-based utilities. Ensure that your contract address is securely stored and consider verifying the source code on Cronoscan for transparency and trust.
For further development ideas, explore our Einstein Contract on GitHub, which includes advanced features and modular contract patterns.
Be the first to leave a comment.